Suppose we have the following NQE query, which takes a List of Strings as a parameter called “awsRegion”. This NQE query returns a list of all NAT Gateways in AWS that are in a region in the given list.
@query
natGatewaysByRegion(awsRegion : List<String>) =
foreach cloudAccount in network.cloudAccounts
where cloudAccount.cloudType == CloudType.AWS
foreach vpc in cloudAccount.vpcs
foreach natGateway in vpc.natGateways
where natGateway.region in awsRegion
select { vpcId: vpc.id, natGatewayId: natGateway.id, region: natGateway.region };
In the GUI, you click the Parameters button, enter the region names, click save, and then execute.
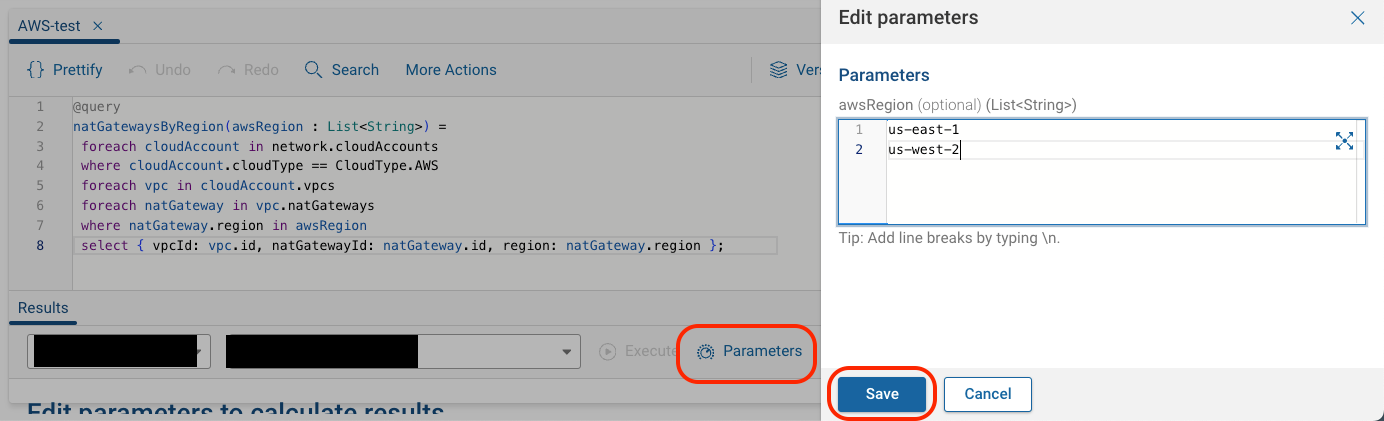
You can perform this same NQE query from the API.
Using the curl command and entering a list of regions with s“us-east-1”, “us-east-2”] as the parameter.
curl -X 'POST' \
'https://fwd.app/api/nqe?networkId=123123' \
-H 'accept: application/json' \
-H 'Authorization: Basic <redacted>' \
-H 'Content-Type: application/json' \
-d '{
"queryId": "Q_1234567891011121231415161718192021",
"parameters": {
"awsRegion": "us-east-1", "us-east-2"]
}
}'
Note the network ID is required in the URL, and a queryId is required to specify what NQE query is being run.